02. 자바스크립트(JavaScript) ES5 기본
OS | Windows 10 Home 64bit 버전 1903 (OS 빌드 18362.836) |
JavaScript | ES5 (2009年) |
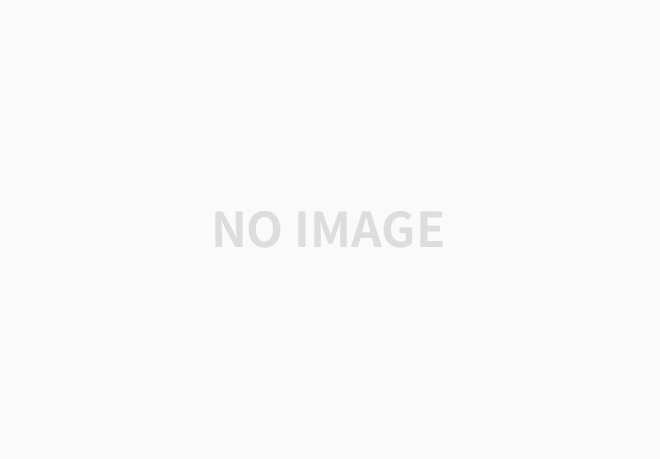
# 간단 설명
- 배열에 forEach, map, filter, reduce, some, every와 같은 메소드 지원
- Object에 대한 getter / setter 지원
- 자바스크립트 strict 모드 지원
- JSON 지원 ( 과거에는 XML을 사용하다가, json이 뜨면서 지원하게 됨 )
- 웹 표준에 적합하다
- ES6은 마이크로소프트 익스플로러 (Microsoft exploror) 낮은 버전에서는 지원하지 않기 때문이다.
# 온라인 에디터
PlayCode - Javascript Playground
Run javascript or typescript code online and see the result as you type. Best for practice code and developing complex algorithms
playcode.io
#0. 함수
함수 선언식과 함수 표현식이 존재한다.
함수 선언식 | 함수 표현식 |
![]() |
![]() |
함수 표현식은 호이스팅의 영향을 받지 않는다 | |
유연함 | 클로저로 사용 |
콜백으로 사용 (다른 함수의 인자로 넘길 수 있음) |
참고
함수 표현식 vs 함수 선언식
(기본) 자바스크립트 함수 표현식과 함수 선언식에는 어떠한 차이점이 있는지 알아봅니다.
joshua1988.github.io
#1. 객체생성
생성자 함수 객체 생성
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
// 생성자 함수 (Constructor function)
function Member(name, country) {
this.name = name;
this.country = country;
this.information = function() {
console.log('이름은 ' + this.name + ' 국가는 ' + this.country + ' 입니다.');
}
}
var member = new Member('heydaze', 'korea');
console.log(member.name);
console.log(member.country);
console.log(member.information());
|
cs |
리터럴 함수 객체 생성
1
2
3
4
5
6
7
8
9
10
11
12
13
|
// 리터럴 함수 (literal function) = 익명 함수 (Anonymous function)
var member = {
name: 'heydaze',
country: 'korea',
information: function() {
console.log('이름은 ' + this.name + ' 국가는 ' + this.country + ' 입니다.');
}
}
console.log(member.name);
console.log(member.country);
console.log(member.information());
|
cs |
오브젝트 생성자 객체 생성
1
2
3
4
5
6
7
8
9
10
11
12
|
// Object 객체 생성
var user = new Object();
user.name = 'heydaze';
user.country = 'korea';
user.information = function() {
console.log('이름은 ' + this.name + ' 국가는 ' + this.country + ' 입니다.');
}
console.log(user.name);
console.log(user.country);
console.log(user.information());
|
cs |
오브젝트.create() 객체 생성
방법 1
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
|
// 생성자 함수 (Constructor function)
function Member() {}
// Object.create 객체 생성
var user = Object.create(Member.prototype, {
name: {
value: 'heydaze'
},
country: {
value: 'korea'
},
information: {
value: function() {
console.log('이름은 ' + this.name + ' 국가는 ' + this.country + ' 입니다.');
}
}
});
console.log(user.name);
console.log(user.country);
console.log(user.information());
|
cs |
방법 2
1
2
3
4
5
6
7
8
9
10
11
12
13
14
|
function Square(width, height) {
this.width = width;
this.height = height;
}
Square.prototype = {
info: function() {
console.log(this.width * this.height);
}
}
var square = new Square(3,5);
square.info();
|
cs |
클래스 객체 생성
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
|
// 클래스(class) 객체생성
class Member {
constructor(name, country) {
this.name = name;
this.country = country;
}
information() {
console.log('이름은 ' + this.name + ' 국가는 ' + this.country + ' 입니다.');
}
}
var member = new Member('heydaze', 'korea');
console.log(member.name);
console.log(member.country);
console.log(member.information());
|
cs |
방식 | 값 | 크롬 콘솔 |
생성자 |
![]() |
![]() |
리터럴 | ![]() |
![]() |
오브젝트 생성자 | ![]() |
![]() |
오브젝트.create |
![]() |
![]() |
![]() |
![]() |
|
클래스 (ES6) |
![]() |
![]() |
#2. 메소드 추가
생성자 함수 Member 에 메소드 추가
방법 1
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
|
// 생성자 함수 (Constructor function)
function Member(name, country) {
this.name = name;
this.country = country;
this.information = function() {
console.log('이름은 ' + this.name + ' 국가는 ' + this.country + ' 입니다.');
}
}
var member = new Member('heydaze', 'korea');
console.log(member.name);
console.log(member.country);
console.log(member.information());
// 메소드 추가 (상속의 개념)
Member.prototype.greeting = function() {
console.log('안녕하세요 ' + this.name + ' 입니다');
}
var member = new Member('heydaze', 'korea');
console.log(member.greeting());
|
cs |
방법 2
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
|
// 생성자 함수 (Constructor function)
function Member(name, country) {
this.name = name;
this.country = country;
this.information = function() {
console.log('이름은 ' + this.name + ' 국가는 ' + this.country + ' 입니다.');
}
}
var member = new Member('heydaze', 'korea');
console.log(member.name);
console.log(member.country);
console.log(member.information());
// 메소드 추가
Member.prototype = Object.create({
greeting:function() {
console.log('안녕하세요 ' + this.name + ' 입니다')
}
});
var member = new Member('heydaze', 'korea');
console.log(member.greeting());
|
cs |
값 | 크롬 콘솔 |
![]() |
![]() |
var memer 가 아닌 function Member 에 메소드가 추가되었다.
메소드 추가 하기 이전에 객체가 생성되어 새롭게 추가된 메소드는 불러오지 못하기 때문에
다시 객체를 생성해야만 greeting() 메소드를 사용할 수 있다.
인스턴스화 된 객체 member에 메소드 추가
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
|
// 생성자 함수 (Constructor function)
function Member(name, country) {
this.name = name;
this.country = country;
this.information = function() {
console.log('이름은 ' + this.name + ' 국가는 ' + this.country + ' 입니다.');
}
}
var member = new Member('heydaze', 'korea');
console.log(member.name);
console.log(member.country);
console.log(member.information());
// 메소드 생성
member.greeting = function() {
console.log('안녕하세요 ' + this.name + ' 입니다');
}
console.log(member.greeting())
|
cs |
값 | 크롬 콘솔 |
![]() |
![]() |
function Member 가 아닌 var member 에만 메소드가 추가 되었다.
리터럴 함수에 메소드 추가
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
|
// 리터럴 함수 (literal function) = 익명 함수 (Anonymous function)
var member = {
name: 'heydaze',
country: 'korea',
information: function() {
console.log('이름은 ' + this.name + ' 국가는 ' + this.country + ' 입니다.');
}
}
// 메소드 추가
member.greeting = function() {
console.log('안녕하세요 ' + this.name + ' 입니다');
}
console.log(member.greeting());
|
cs |
#3. 프로퍼티, 프로퍼티 속성
자바스크립트는 이름과 속성을 갖춘 프로퍼티의 집합이다.
프로퍼티의 속성 2가지
데이터 프로퍼티 | 설명 |
value | 모든 자료형 가능 |
writable | 데이터 프로퍼티 변경 가능 여부 정의 |
enumerable | for-in 구문 사용가능 여부 |
configurable | 제거, 접근프로퍼티 변경여부, 쓰기 불가여부, enumerable 속성 수정 가능 여부 |
접근 프로퍼티 | 설명 |
get 접근자 | Function 혹은 undefined 지정 |
set 접근자 | Function 혹은 undefined 지정 |
enumerable | for-in 구문 사용가능 여부 |
configurable | 제거, 데이터프로퍼티 변경, 다른 속성들의 수정 가능 여부 지정 |
프로퍼티 접근 방법 2가지
점 표기법 | 대괄호 표기법 |
member.name | member['name'] |
![]() |
![]() |
![]() |
![]() |
![]() |
![]() |
![]() |
![]() |
writable
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
|
// 생성자 함수 (Constructor function)
function Member(name, country) {
this.name = name;
this.country = country;
this.information = function() {
console.log('이름은 ' + this.name + ' 국가는 ' + this.country + ' 입니다.');
}
Object.defineProperty(this, 'name', {
value: name,
writable: false,
enumerable: false,
configurable: false
});
};
var member = new Member('heydaze', 'korea');
// writable = true
console.log('before=', member.country)
member.country = 'japan';
console.log('after=', member.country);
console.log('-------------------')
// writable = false
console.log('before=', member.name);
member.name = 'star';
console.log('after=', member.name);
|
cs |
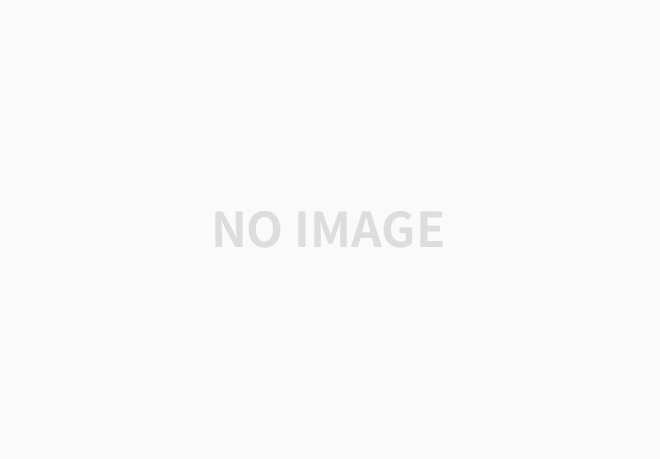
enumerable
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
|
// 생성자 함수 (Constructor function)
function Member(name, country) {
this.name = name;
this.country = country;
this.information = function() {
console.log('이름은 ' + this.name + ' 국가는 ' + this.country + ' 입니다.');
}
}
// 인스턴스화
var member = new Member('heydaze', 'korea');
// enumerable=true
for (var prop in member) {
console.log('prop=', prop);
console.log('val=', member[prop]);
console.log();
}
|
cs |
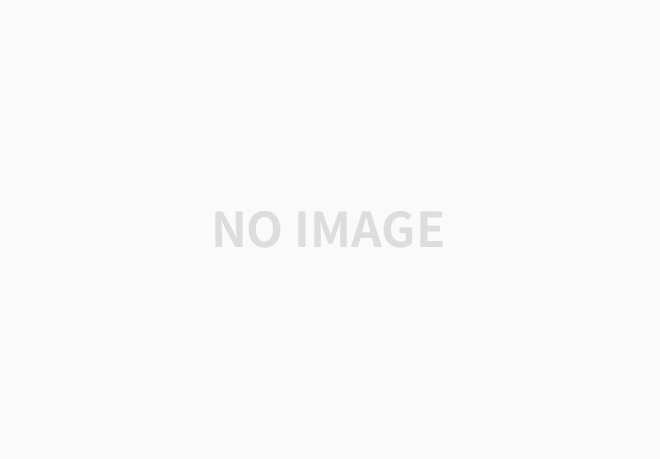
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
|
// 리터럴 함수 (literal function) = 익명 함수 (Anonymous function)
var member = {
name: 'heydaze',
country: 'korea',
information: function() {
console.log('이름은 ' + this.name + ' 국가는 ' + this.country + ' 입니다.');
}
}
// enumerable=true
for (var prop in member) {
console.log('prop=', prop);
console.log('val=', member[prop]);
console.log();
}
|
cs |
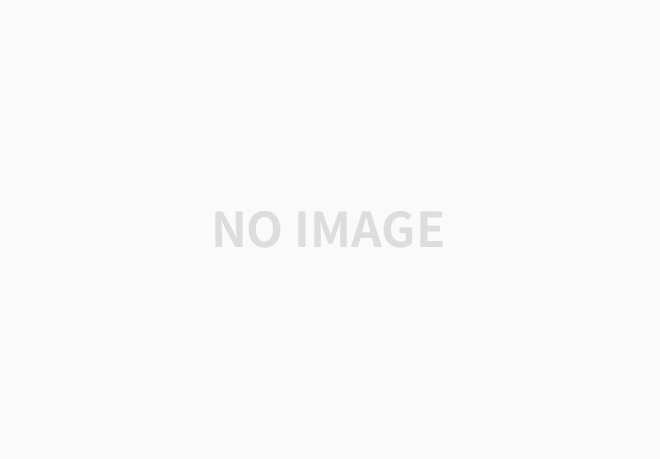
1
2
3
4
5
6
7
8
9
10
|
// Array
var animals = ['dog', 'cat', 'horse'];
// enumerable=true
for (var animal in animals) {
console.log('index=', animal);
console.log('val=', animals[animal]);
console.log();
}
|
cs |
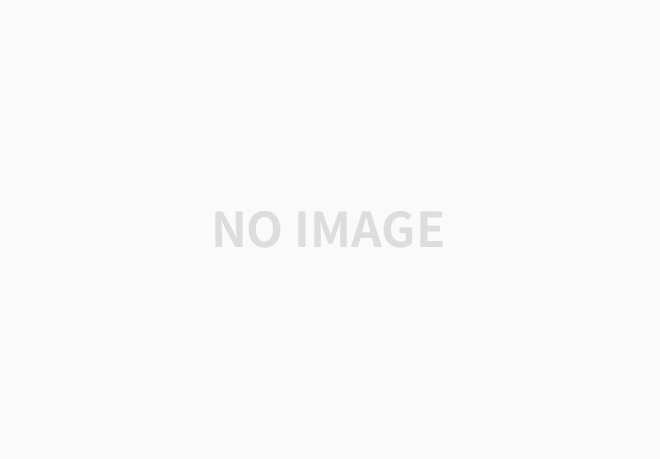
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
|
// 생성자 함수 (Constructor function)
function Member(name, country) {
this.name = name;
this.country = country;
this.information = function() {
console.log('이름은 ' + this.name + ' 국가는 ' + this.country + ' 입니다.');
}
Object.defineProperty(this, 'name', {
value: name,
writable: false,
enumerable: false,
configurable: false
});
};
var member = new Member('heydaze', 'korea');
// enumerable (name=false)
for (var prop in member) {
console.log('prop=', prop);
console.log('val=', member[prop]);
console.log
}
|
cs |
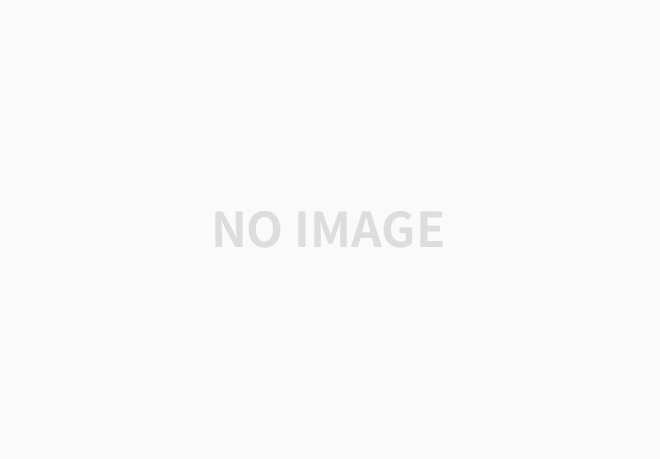
구조보기
Object.defineProperty 는 프로퍼티를 추가, 수정 할 수 있다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
|
// 생성자 함수 (Contructor function)
function Member(name, country) {
var _name = name;
var _country = country;
// 데이터 프로퍼티 추가
Object.defineProperty(this, 'name', {
value: _name,
writable: true,
enumerable: true,
configurable: false
});
// 데이터 프로퍼티 추가
Object.defineProperty(this, 'country', {
value: _country,
writable: true,
enumerable: true,
configurable: false
});
// 접근자 프로퍼티 추가
Object.defineProperty(this, 'information', {
get: function() {
console.log('이름은 ' + _name + ' 국가는 ' + _country + ' 입니다.');
},
set: function(newValue) {
return newValue;
},
enumerable: true,
configurable: false
});
// 데이터 프로퍼티 추가
Object.defineProperty(this, 'greeting', {
value: function() {
console.log('안녕하세요 ' + _name + ' 입니다');
},
writable: true,
enumerable: true,
configurable: false
});
};
// 인스턴스화
var member = new Member('heydaze', 'korea');
console.log(member.name);
console.log(member.country);
console.log(member.information); // 함수 X
console.log(member.greeting()); // 함수 O
|
cs |
값 | 크롬 콘솔 |
![]() |
![]() |
#4. 프로토 (__proto__)
상속 확인하는 방법
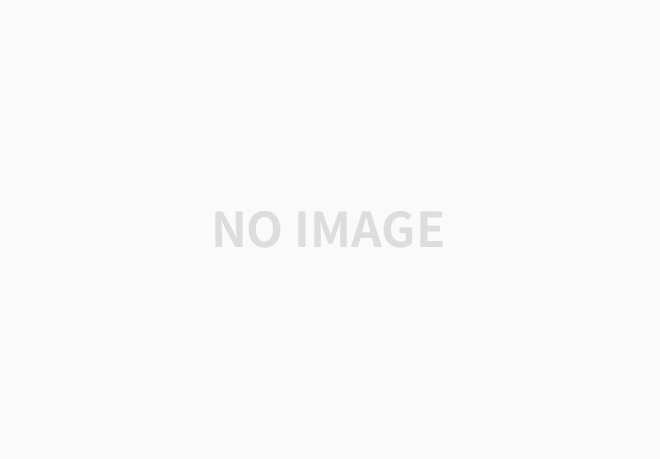
instanceof 를 이용하여 상속되어있는지 확인이 가능하지만,
바로 위에 상속된 객체가 누구인지 확인하려면 위 방법이 좋다.
추가 & 재정의
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
|
// 생성자 함수
function Func() {
this.a = 1;
this.b = 2;
}
// 인스턴스화
var obj = new Func();
// 프로토타입에 b와 c 추가
Func.prototype.b = 3;
Func.prototype.c = 4;
// 출력
console.log(obj.a);
console.log(obj.b);
console.log(obj.c);
console.log(obj.d);
|
cs |
값 | 크롬 콘솔 |
![]() |
![]() |
__proto__ 는 자바에서 상위 클래스라고 생각하면 된다.
b는 재정의 되어 3이 아닌 2가 되고 d는 존재 하지않아 undefined 를 반환한다.
proto의 b에 접근하려면 obj.__proto__.b 로 작성하면 된다.
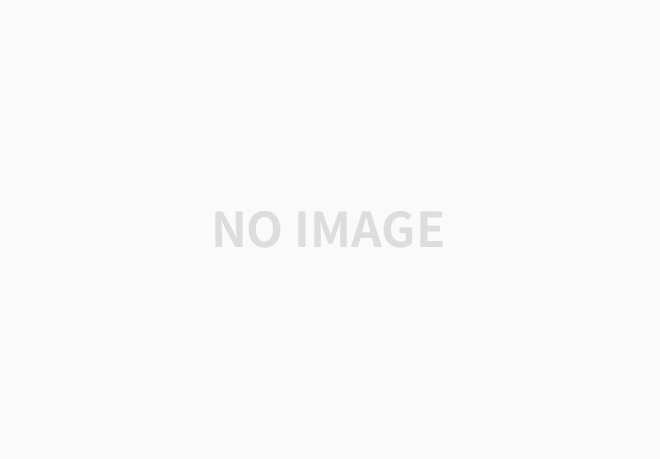
메소드 상속
1
2
3
4
5
6
7
8
9
10
11
12
13
|
var obj = {
a: 2,
m: function(b) {
return this.a+1;
}
}
console.log(obj.m()); // 2+1
var obj2 = Object.create(obj);
obj2.a = 12;
console.log(obj2.m()); // 12+1
|
cs |
Object.create(obj) 를 통해 obj 를 상속받는 객체 obj2 를 생성한다.
출력 | |
![]() |
|
obj | ![]() |
obj2 | ![]() |
obj2 객체에는 m 이라는 메소드가 존재하지 않지만
__proto__ 에 m 이라는 메소드가 존재하여 사용할 수 있다.
m 이외에도 거의 모든 객체에는 Object를 상속받기 때문에 Object의 메소드들을 사용할 수 있다.
클래스 상속 (ES6)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
|
// 부모 클래스
class Polygon {
// 생성자
constructor(width, height) {
this.width = width;
this.height = height;
}
// getter
get area() {
return 'area=' + (this.width * this.height);
}
}
// 자식 클래스
class Square extends Polygon {
// 생성자
constructor(sideLength) {
// 부모 클래스 생성자
super(sideLength, sideLength);
}
// setter
set sideLength(newLength) {
this.width = newLength;
this.height = newLength;
}
}
// 인스턴스화
var square = new Square(5);
console.log(square.area); // area=25 (getter)
square.sideLength = 3; // width=3,height=3 (setter)
console.log(square.area); // area=9 (getter)
|
cs |
값 | 크롬 콘솔 |
![]() |
![]() |
추가 예제
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
|
// 생성자 함수 (Constructor function)
function Member(name, country) {
this.name = name;
this.country = country;
} // Member {name:'heydaze', country:'korea'}
// __proto__에 메소드 추가
Member.prototype.information = function() {
console.log('이름은 ' + this.name + ' 국가는 ' + this.country + ' 입니다.');
} // Member {name:'heydaze', country'korea', __proto__: information()}
// 생성자 함수 (Constructor function)
function TeamMember(name, country, task) {
Member.call(this, name, country);
this.task = task;
} // TeamMember(name:'heydaze', country:'korea', task:['cook', 'fishing'], __proto__: constructor:Member)
TeamMember.prototype = Object.create(Member.prototype);
// TeamMember(name:'heydaze', country:'korea', task:['cook', 'fishing'], __proto__: __proto__: information(), constuctor:Member)
TeamMember.prototype.greeting = function() {
console.log('안녕하세요 ' + this.name + ' 입니다');
} // TeamMember(name:'heydaze', country:'korea', task:['cook', 'fishing'], __proto__: greeting(), __proto__: information(), constuctor:Member)
TeamMember.prototype.work = function() {
console.log('task_length=', this.task.length);
} // TeamMember(name:'heydaze', country:'korea', task:['cook', 'fishing'], __proto__: greeting(), work(), __proto__: information(), constuctor:Member)
var member = new TeamMember('heydaze', 'korea', ['cook', 'fishing']);
console.log(member.name);
console.log(member.country);
console.log(member.information());
console.log(member.greeting());
console.log(member.work());
|
cs |
값 | 크롬 콘솔 |
![]() |
![]() |
프로퍼티 체인을 확인 할 때에는
TeamMember.prototype.isPrototypeOf(member);
#5. 스코프와 클로저
스코프는 변수의 접근성에 관한 것이다.
# | 종류 |
1 | 클래스-레벨 스코프(class-level scope) |
2 | 메소드-레벨 스코프(method-level scope) |
3 | 블록-레벨 스코프(block-level scope) |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
|
// 스코프와 클로저
function bookHotel (city) {
var availableHotel = 'None';
for (var i=0; i<hotels.length; i++) {
var hotel = hotels[i];
if (hotel.city === city && hotel.hasRoom) {
availableHotel = hotel.name;
break;
}
}
// 여기서 i와 hotel은 여전히 접근 가능하다
console.log('Checked ' + (i+1) + ' record(s)'); // Checked 2 record(s) 출력
console.log('Last checked ' + hotel.name); // Last checked Hotel B 출력
{
function placeOrder() {
var totalAmount = 200;
console.log('Order placed to ' + availableHotel);
}
}
placeOrder();
// 접근 불가
// console.log(totalAmount);
return availableHotel;
}
var hotels = [{name: 'Hotel A', hasRoom: false, city: 'Sanya'},
{name: 'Hotel B', hasRoom: true, city: 'Sanya'}];
console.log(bookHotel('Sanya')); // Hotel B 출력
// 접근 불가
// console.log(availableHotel);
|
cs |
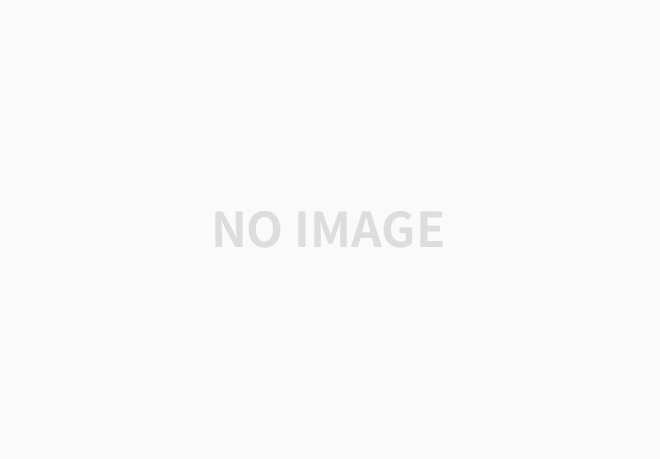
var hotels 변수는 전역 스코프 (전역변수)
var availableHotel 변수는 bookHotel() 함수의 스코프 (로컬변수)
availableHotel 는 bookHoel() 어디에서나 접근이 가능하다.
중첩된 placeOrder() 함수에도 접근이 가능한 데 여기서 중첩된 함수를 클로저(closure) 라 부른다.
클로저는 함수가 또 다른 함수 내부에 중첩된 형태를 이룬다.
var totalAmount 변수는 placeOrder() 함수의 로컬변수 이다
#6. this
자바스크립트에서 this 는 현재 실행 컨텍스트(cuurent execution context)를 참조하며,
이 실행 컨텍스트도 하나의 객체다
실행 코드 | 설명 |
전역코드 | 자바스크립트 프로그램이 시작되는 곳부터 수행되는 코드다. 브라우저의 경우에는 window 객체가 존재한다. 그리고 브라우저 콘솔을 열고 var user = new User() 를 입력하면 전역 코드를 작성한 것 이다. |
Eval 코드 | 내장된 eval() 함수의 인자로 전달되는 문자열 값이다 (실행할 작업에 대해 정확히 모른다면 eval() 함수를 사용하지 말아야 한다) |
함수 코드 | 함수의 본문코드다. 하지만 함수 내부에 작성된 모든 코드가 함수 코드를 의미하지는 않는다. |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
|
function User (name) {
console.log('1. I\'m in "' + this.constructor.name + '" context.'); // User 컨텍스트
this.name = name;
this.speak = function () {
console.log('2. ' + this.name + ' is speaking from "' + this.constructor.name + '" context.'); // User 컨텍스트
var drink = function () {
console.log('3. Drinking in "' + this.constructor.name + '"'); // Window 컨텍스트
}
drink();
};
function ask() {
console.log('4. Asking from "' + this.constructor.name + '" context.'); // Window 컨텍스트
console.log('5. Who am I? "' + this.name + '"'); // Window 컨텍스트 에는 name 이 존재 하지 않는다
}
ask();
}
var name = 'Unknown';
var user = new User('Ted');
user.speak();
|
cs |
값 | 크롬 콘솔 |
![]() |
![]() |
자바스크립트 엔진이 함수 선언을 발견하면 function 객체를 생성하며
이 객체는 함수가 선언된 스코프 내에서 접근 가능하다.
var drink() 변수는 User 객체의 프로퍼티가 아니다.
따러서 speak() 메소드 안에서만 접근이 가능하다
speak() 메소드와 ask 함수는 같은 스코프에 있지만 서로 다른 실행 컨텍스트를 가진다.
[객체 인스턴스화]
전역 스코프 -> User 함수 스코프
[ask 함수]
User 함수 스코프 -> ask 함수 스코프
ask 함수는 객체의 프로퍼티도 아니며, ask.call(this) 로 호출되지 않았음으로 전역 컨텍스트 이다.
ask.call(this) 를 이용하여 컨텍스트를 바꿀 수 있다.
[speak() 메소드 호출]
전역 스코프 -> User 함수 스코프
호출 방법 | 코드 |
생성자 함수 호출 | new User(); |
직접 함수 호출 | ask(); |
메소드 호출 | user.speak(); |
컨텍스트 변경 호출 | ask.call(this) 또는 ask.apply(this) |
#7. 호이스팅
호이스팅은 자바스크립트의 인터프리터가 함수 선언과 변수 선언들을
선언들이 속해 있는 스코프의 최상단으로 끌어올리는 것을 말한다.
예제 1
작성 코드 | 인터프리터 코드 |
![]() |
![]() |
1순위 함수선언 |
|
2순위 변수선언 | |
3순위 그 외 | |
travel 함수를 선언했지만 그 다음 변수로 travel = '계획 없음' 으로 변경되었기 때문에 console.log(travel); 값은 '계획없음' 이 출력되고, travel() 함수는 사라졌다. |
예제 2
작성 코드 | 인터프리터 코드 |
![]() |
![]() |
return; 에서 끝나야 되는데 'Gym B' 가 출력되는 것으로 goToGym() 함수는 사용되는 것을 확인 할 수 있다. | |
함수선언식은 호이스팅의 영향을 받지만, 함수표현식은 호이스팅에 영향을 받지 않는다. |
|
때문에 goToGym() 함수가 var goToGym 메소드 위에 있는 것이다. |
'06. Javascript > 기초' 카테고리의 다른 글
11. 자바스크립트 (JavaScript) - 2개이상의 obj 합치기 ( Object.assign ) (0) | 2021.06.09 |
---|---|
08. 자바스크립트 (JavaScript) - Console (0) | 2020.09.23 |
06. 자바스크립 (JavaScript) ES5 의 CallBack 과 ES6의 Promise (0) | 2020.08.15 |
04. 자바스크립트(JavaScript) ES5, ES6 Array [미완성] (0) | 2020.07.27 |
03. 자바스크립트(JavaScript) ES6 기본 (0) | 2020.07.27 |
댓글
이 글 공유하기
다른 글
-
08. 자바스크립트 (JavaScript) - Console
08. 자바스크립트 (JavaScript) - Console
2020.09.23 -
06. 자바스크립 (JavaScript) ES5 의 CallBack 과 ES6의 Promise
06. 자바스크립 (JavaScript) ES5 의 CallBack 과 ES6의 Promise
2020.08.15 -
04. 자바스크립트(JavaScript) ES5, ES6 Array [미완성]
04. 자바스크립트(JavaScript) ES5, ES6 Array [미완성]
2020.07.27 -
03. 자바스크립트(JavaScript) ES6 기본
03. 자바스크립트(JavaScript) ES6 기본
2020.07.27
댓글을 사용할 수 없습니다.